What is an interface? It’s a key feature in object-oriented programming and yet so mysterious to developers getting their start in the world of software development. Quite simply, an interface is a language specific representation of a contract used in computer programming languages. Interfaces cannot be instantiated, and they cannot define implementations. They are just a list of definitions for properties and methods that are exposed by a class that implements this interface.
Interfaces in object-oriented software development
Since an interface represents a contract, the invoking object doesn’t need to know anything about the implementation of the called object. It just needs to understand the contract they share. Look at this example in C#.
interface IWriter
{
void Write(Paper paper, string sentence);
}
In C# interface names typically start with the letter ‘I’. In this case, our interface is named IWriter and exposes a method called Write that takes a type Paper named paper and a string called sentence as parameters, and returns type void. This is pretty descriptive of what the contract is. An object that implements this interface will write the sentence to the paper. As a software developer, you don’t need to know anything else about the type you are calling into and it’s implementation.
How do we implement an interface?
Interfaces are easy to implement. You inherit from the interface and define all of the methods and properties the interface demands of you.
class PaperWriter : IWriter
{
public void Write(Paper paper, string sentence)
{
paper.Lines.AddText(sentence);
paper.Flush();
}
}
You’ll see in our example, we define our class named PaperWriter and implement the IWriter interface. Then we define the Write method by adding the sentence to the paper Lines property by calling a method AddText. After this, we call Flush on the paper object to Flush the text from any buffers that the object holds and we are done.
Software development with interfaces
Once we have defined our interface and we have implemented it, it is now ready to be used by a calling object. One way to utilize the interface is by using a Factory pattern to create the object so we get the right implementation.
static class PaperWriterFactory
{
public static IWriter CreateWriter()
{
return new PaperWriter();
}
}
class Program
{
static void Main(string[] args)
{
IWriter writer = PaperWriterFactory.CreateWriter();
Paper paper = new Paper();
writer.Write(paper, "Hello World!");
Console.WriteLine(paper.Text);
Console.ReadLine();
}
}
Build better software
Now that we have a good feel for how the interface works and how it plays out, let’s include the code we left out. Namely, this will be the definitions for Paper and another class called Lines.
class Paper
{
public Paper()
{
Lines = new Lines();
}
public string Text { get; private set; }
public Lines Lines { get; }
public void Flush()
{
StringBuilder builder = new StringBuilder(Lines.CharacterCount);
foreach (var line in Lines.ToArray())
{
builder.Append(line);
}
Text = builder.ToString();
}
}
class Lines
{
private readonly List<string> _lines = new List<string>();
public int CharacterCount { get; private set; }
public string[] ToArray()
{
return _lines.ToArray();
}
public void AddText(string line)
{
CharacterCount += line.Length;
_lines.Add(line);
}
}
You’ll see these two objects are fairly simple and straightforward. Paper initializes a Lines property in the constructor and also has a property for Text. Then Paper also implements the Flush method we saw earlier by taking whatever is stored in Lines and converting that to the Text string property.
Lines contains all of the lines that have been added to the Paper object. It also has a property CharacterCount and a method ToArray that both help with the flushing the buffer later.
When the program runs, you’ll see this on your console:
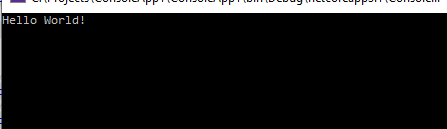
That is it! You now know what you need to start using interfaces in your software development going forward. Happy coding!
Read about our Software Development service and read a case study about how we were able to help a small business with our services.